[CTF] WU - Layered Mystery
CTF InterCampus Ynov 2024
Difficulty Level : Hard
Challenge Category : Misc
Description :
Dive deep through multiple layers to uncover hidden secrets. Use clues and evidence from the past to navigate and solve the puzzles. Each layer reveals new challenges—how far can you go?
PS: first password is oxyhack
Solution Steps
Overview
This challenge involves:
- A series of zip files nested within folders, each protected by a password.
- XOR-based computations to derive the password for the next layer.
- Locating a
flag.txt
file hidden within one of the extracted layers.
Step-by-step Solution
1. Initial Setup
- Start with the provided zip file and the first password (
oxyhack
). - Extract the first zip file to reveal additional layers.
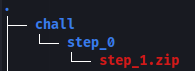
2. Understand the Puzzle Logic
- Each step involves:
- Extracting a zip file using a password.
- Locating a text file containing a hex-encoded string.
- Computing the password for the next step using the XOR operation.
Python Script Explanation
The provided script automates the solution for this challenge. Below are its components:
2.1 XOR Computation
The xor_hex_string
function decodes a hex string, XORs it with the current password, and computes the password for the next layer.
def xor_hex_string(hex_string, key):
if isinstance(key, str):
key = key.encode('utf-8')
return xor(bytes.fromhex(hex_string.strip()), key).decode()
2.2 Zip File Extraction
The extract_zip
function extracts a zip file using the given password. It validates successful extraction or stops the process if an error occurs.
def extract_zip(zip_path, extract_to, password):
command = f'unzip -P "{password}" -d "{extract_to}" "{zip_path}"'
result = subprocess.run(command, shell=True, capture_output=True)
if result.returncode != 0:
print(f"Error while extracting {zip_path}: {result.stderr.decode()}")
sys.exit(1)
2.3 Flag Search
The find_flag
function recursively searches the extracted folder for a flag.txt
file. If found, it prints the flag and terminates the process.
def find_flag(base_folder):
for root, _, files in os.walk(base_folder):
if 'flag.txt' in files:
flag_path = os.path.join(root, 'flag.txt')
with open(flag_path, 'r') as f:
print("[+] Flag found!")
print(f"Flag content: {f.read()}")
return True
return False
2.4 Challenge Solver
The solve_challenge
function orchestrates the solution:
- Extracts each zip file using the current password.
- Reads the hex-encoded string for the next password.
- Computes the password using XOR.
- Repeats until the
flag.txt
file is found.
def solve_challenge():
base_folder = 'chall'
password = "oxyhack"
step = 0
initial_dir = os.getcwd()
while True:
zip_path = os.path.join(initial_dir, f"{base_folder}/step_{step}/step_{step+1}.zip")
if not os.path.exists(zip_path):
print(f"The file {zip_path} does not exist. Stopping.")
break
print(f"[+] Extracting {zip_path} with the password: {password}")
extract_zip(zip_path, initial_dir, password)
extracted_folder = os.path.join(initial_dir, base_folder, f"step_{step + 1}")
if not os.path.exists(extracted_folder):
print(f"The folder {extracted_folder} is missing after extraction. Stopping.")
break
if find_flag(extracted_folder):
break
hex_file = os.path.join(extracted_folder, f"step_{step + 2}.txt")
if not os.path.exists(hex_file):
print(f"The file {hex_file} is missing. Stopping.")
break
with open(hex_file, 'r') as f:
hex_string = f.read().strip()
print(f"[+] Computing the password for step_{step + 1}...")
password = xor_hex_string(hex_string, password)
print(f"[+] Password found: {password}")
step += 1
2.5 Execution
Run the script to automate the extraction and decryption process:
python solve_challenge.py
Expected Output
- The script iteratively extracts zip files and computes passwords using the XOR logic.
- Upon locating the
flag.txt
file, the script outputs its content:[+] Flag found! Flag content: FLAG{example_flag_here}
